在现代Web开发中,发送电子邮件是许多应用程序的基本功能之一。PHP作为一种广泛使用的服务器端脚本语言,提供了简单而有效的方法来实现电子邮件发送功能。为了更复杂的需求,例如发送带有附件的电子邮件,PHP提供了多种解决方案来实现这一点。在杏耀注册网站建设中,我们将深入探讨如何使用PHP程序发送带附件的邮件,涵盖从最基础的mail()函数到使用功能更为强大的PHPMailer库的方法。
### 使用PHP的mail()函数发送邮件

PHP内置的`mail()`函数提供了基本的发送电子邮件的功能。其语法如下:
```php
bool mail(string $to, string $subject, string $message, string $headers, string $parameters);
```

虽然`mail()`函数简单易用,但它在处理附件方面显得有些局限。为了发送邮件带附件,我们需要手动构建电子邮件的MIME格式。
### 构造MIME邮件以发送附件
要发送带附件的电子邮件,我们需要手动设置邮件的头部信息以支持MIME(Multipurpose Internet Mail Extensions)格式。这允许我们发送非文杏耀注册网站建设件作为附件。以下是一个示例代码展示了如何使用PHP的`mail()`函数发送带附件的邮件:
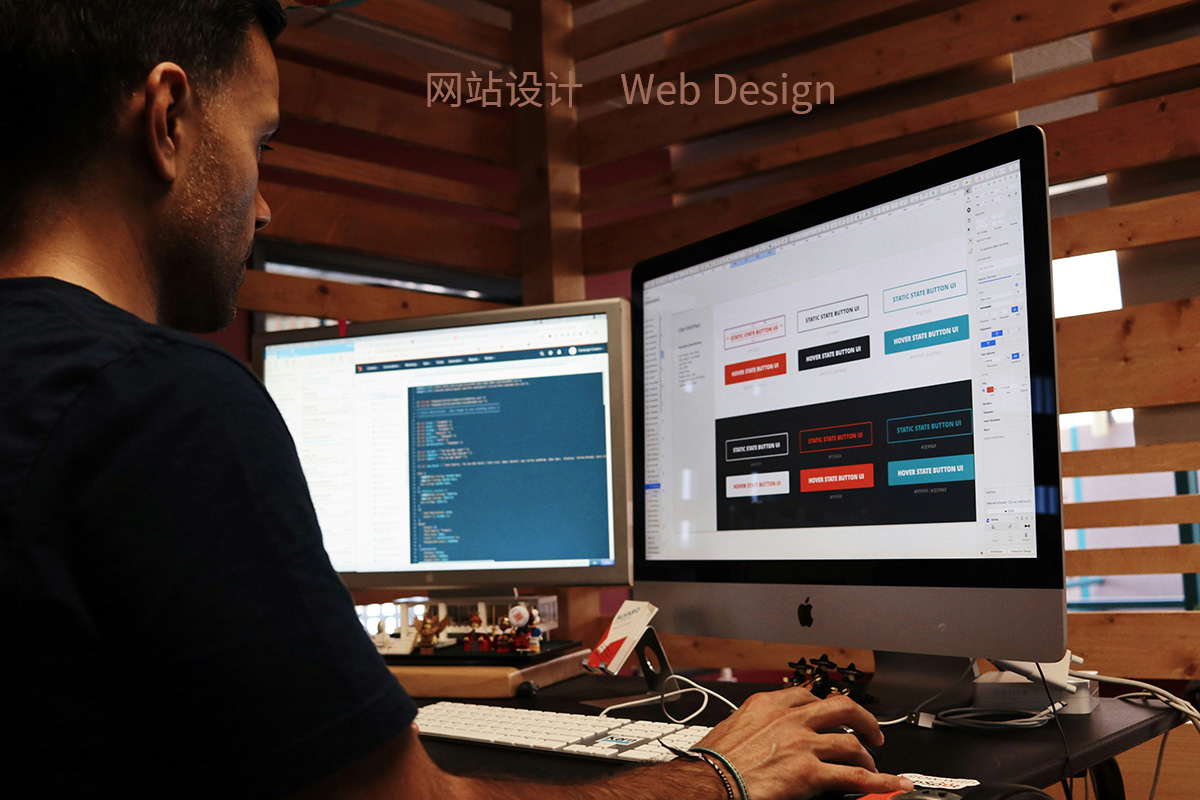
```php
$to = 'recipient@example.com';
$subject = 'Here is your attachment';
$message = 'Please see the attached file.';

$file = '/path/to/your/file.txt';
$fileData = file_get_contents($file);
$fileType = mime_content_type($file);
$fileName = basename($file);

$boundary = md5(time());
$headers = "MIME-Version: 1.0\r\n";
$headers .= "From: sender@example.com\r\n";
$headers .= "Reply-To: sender@example.com\r\n";
$headers .= "Content-Type: multipart/mixed; boundary=\"$boundary\"";

$body = "--$boundary\r\n";
$body .= "Content-Type: text/plain; charset=UTF-8\r\n";
$body .= "Content-Transfer-Encoding: 7bit\r\n";
$body .= "\r\n";
$body .= $message . "\r\n";

$body .= "--$boundary\r\n";
$body .= "Content-Type: $fileType; name=\"$fileName\"\r\n";
$body .= "Content-Disposition: attachment; filename=\"$fileName\"\r\n";
$body .= "Content-Transfer-Encoding: base64\r\n";
$body .= "\r\n";
$body .= chunk_split(base64_encode($fileData)) . "\r\n";
$body .= "--$boundary--";
mail($to, $subject, $body, $headers);
?>
```
在这份示例代码中,我们手动构建了邮件消息和附件的MIME边界,并对附件进行了Base64编码以适合邮件传输。这种方法虽然可以实现功能,但代码复杂且不易维护。因此,我们推荐使用更现代化的库,如PHPMailer以简化流程。
### 使用PHPMailer发送带附件的邮件
PHPMailer是一个广受欢迎的PHP邮件发送库,它简化了邮件发送过程并支持各种附加功能,如SMTP、HTML邮件格式、附件等。下面是如何用PHPMailer发送带附件邮件的步骤:
1. **安装PHPMailer**:我们可以使用Composer来安装PHPMailer,这是管理PHP依赖项的首选方法。运行以下命令来安装:
```bash
composer require phpmailer/phpmailer
```
2. **发送邮件代码示例**:
```php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'vendor/autoload.php';
$mail = new PHPMailer(true);
try {
//服务器设置
$mail->SMTPDebug = 2;
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = 'your-email@example.com';
$mail->Password = 'your-email-password';
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587;
//收件人设置
$mail->setFrom('from@example.com', 'Mailer');
$mail->addAddress('recipient@example.com', 'Joe User');
//附件
$mail->addAttachment('/path/to/file.txt');
//内容
$mail->isHTML(true);
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body
in bold!';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
?>
```
在这个例子中,PHPMailer's强大功能显而易见。我们只需进行简短的配置,就能实现复杂的邮件发送需求。PHPMailer通过内置的SMTP支持和自动处理MIME,使得发送邮件带附件变得更加高效、快捷。
### 为什么选择PHPMailer
1. **易用性**:PHPMailer's接口设计简洁流畅,无需手动编写复杂的邮件头信息。
2. **功能丰富**:支持SMTP、安全连接、HTML格式、附件、多语言等众多功能。
3. **社区支持**:PHPMailer有一个活跃的开发社区,提供快速的更新和支持。
4. **强大的错误处理**:PHPMailer通过异常处理机制提供详细的错误信息,实现更好的错误管理。
### 总结
发送带附件的电子邮件是许多应用程序的基本需求。虽然PHP的`mail()`函数足以满足简单的需求,但使用PHPMailer这样的库可以大大简化代码,提高可读性和维护性。通过PHPMailer的使用,开发者可以快速实现复杂的邮件功能,无需过多关注底层实现细节。
不论选择哪种方法,理解邮件发送的基础原理和不同方法的优缺点都将帮助开发者根据项目的具体需求做出最佳的选择。希望杏耀注册网站建设能够为使用PHP程序发送邮件提供一个清晰的指南。